If statement
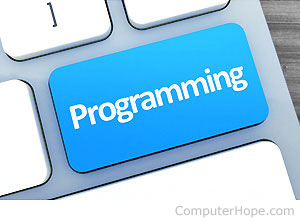
An if statement is a conditional statement in programming that, if true, performs a specified function or displays information on the screen. Below is a general example of an if statement, not specific to any particular programming language.
if (X < 10) { print "Hello John"; }
In the example above, if the value of X equals any number less than 10, the program displays "Hello John" when the script is run.
Examples of if statements
Below are examples of the same if statement above using other programming languages, including C++, Java, JavaScript, Perl, PHP (PHP: Hypertext Preprocessor), Python, Ruby, and Visual Basic.
C#
if (X < 10) { Console.WriteLine("Hello John."); }
C++
if (X > 10) { std::cout << "Hello John." << std::endl; }
Java
if (X < 10) { System.out.println("Hello John."); }
JavaScript & HTML
<p id="example">Display Javascript output here</p> if (X < 10) { document.getElementById("example").innerHTML = "Hello John."; }
Perl
if ($X < 10) { print "Hello John."; }
PHP
if ($X < 10) { echo "Hello John."; }
Python
if X < 0: print("Hello John.")
Ruby
if X < 10 puts "Hello John." end
Visual Basic
If X < 10 Then message = "Hello John." Console.WriteLine(message)
See the conditional statement definition for more information and additional programming examples.
Conditional expression, Else, Elseif, Exists, If else, Logical decision, Programming terms, Statement