Conditional statement
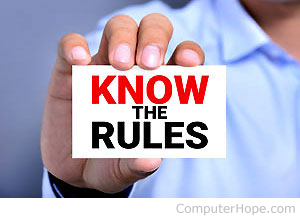
Alternatively known as a conditional expression, conditional flow statement, and conditional processing, a conditional statement is a set of rules performed if a certain condition is met. It is sometimes called an If-Then statement, because IF a condition is met, THEN an action is performed.
For example, consider the following textual example of a conditional statement.
IF a value is less than 10, THEN display the words "Value is less than 10" on the screen.
The example above is how a conditional statement might be interpreted by the human brain. In this example, if the value is true, it performs the action. If it is false, it does nothing.
Using a computer programming language, the conditional statement above could be written like the example statements below.
if ($myval < 10) { print "Value is less than 10"; }
In this example, if the variable $myval is less than 10 ( the expression), then the program prints "Value is less than 10" to the screen.
To make the program do something when a condition is not true an else statement can be added, as shown below.
if ($myval == 10) {
print "The value equals 10";
} else {
print "Value is not equal to 10";
}
In this example, if the variable is exactly equal to 10, it prints "The value equals 10" to the screen. Otherwise, it prints "Myval is not equal to 10" to the screen.
Next, we could add even more conditions by adding an elsif (else if) statement, as shown below.
if ($myval > 10) {
print "Value is greater than 10"; } elsif ($myval < 10) { print "Value is less than 10";
} else {
print "The value equals 10";
}
Finally, this last example prints one of three messages to the screen, depending on if the value is greater than 10, less than 10, or equal to 10.
Boolean, Condition, Else, Else if, Expression, If, If else, If statement, Operator, Programming terms, Statement