Algorithm
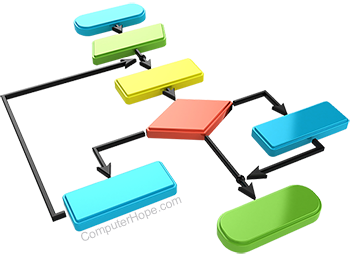
Derived from the name of the mathematician Muhammed ibn-Musa Al-Khowarizmi, an algorithm is a solution to a problem that meets the following criteria.
- A list of instructions, procedures, or formula that solves a problem.
- Can be proven.
- Something that always finishes and works.
Example of an algorithm
The following algorithm counts the number of letters in a word. We first demonstrate the algorithm using pseudocode, which explains the algorithm in an English-like syntax. The same algorithm is shown in a programming language.
Algorithm in pseudocode
Let N = 0 for each letter in the word set N = N + 1
In the example above, the following is happening.
- The number we are counting is declared as starting with 0. In this example, we use the letter "N" as our variable, but it could be anything.
- Start a loop by looking at each letter in the word.
- For each of the letters encountered, increase the count of "N" by one.
Algorithm in programming language
my $word = "hope"; my $n = 0; my @words = split(//, $word); foreach (@words) { $n++; } print "Letters: $n";
In the example above, which is coded in Perl, the following is happening:
- The word "hope" is assigned to the $word variable.
- Our counter is assigned as the $n variable with a starting value of 0.
- The word "hope" is split by letter and each letter is stored in the @words array.
- The foreach loop begins going through each element in the array.
- For each element, the $n counter is increased by one.
- End of the foreach loop that continues to loop while true.
- Print the text "Letters: 4" to the screen because there are four letters in "hope."
How algorithms are used
Today, algorithms are used billions of times every day for various tasks. Below are some ways algorithms are used.
- There are many sort algorithms that sort data.
- Algorithms help control traffic lights.
- Computers use algorithms to convert data (e.g., converting decimal into binary).
- Google's search uses the PageRank algorithm to sort searched results.
- Encryption to encrypt and decrypt information and keep data safe is an algorithm.
- GPS (Global Positioning System) uses graph search algorithms to find the best route to a destination.
- Smartphones, Wi-Fi, and wireless communication use algorithms to communicate.
- E-mail spam detection uses algorithms to filter out bad e-mails.
- Data compression for getting information faster (e.g., YouTube video) use algorithms.
When was the first algorithm?
Because a cooking recipe could be considered an algorithm, the first algorithm could go back as far as written language. However, many find Euclid's algorithm for finding the greatest common divisor to be the first algorithm. This algorithm was first described in 300 B.C.
Ada Lovelace is credited as being the first computer programmer and the first person to develop an algorithm for a machine.
A* algorithm, Bubble, Cipher, Computer science, Crypto terms, Exponential backoff, Flowchart, Hashing, Logical decision, MDC, Monte Carlo Method, Programming terms, Quantum algorithm, Routing algorithm