Bash export builtin command
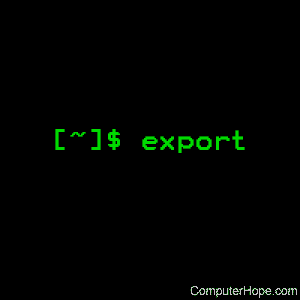
On Unix-like operating systems, export is a builtin command of the Bash shell. It designates specified variables and functions to be passed to child processes.
Description
Normally, when you run a command at the bash prompt, a dedicated process is created with its own environment, exclusively for running your command. Any variables and functions you defined before running the command are not shared with new processes — unless you explicitly mark them with export.
Syntax
export [-f] [-n] [name[=value] ...]
export -p
Options
The export builtin command has the following options:
-f | The -f option must be used if the names refer to functions. If -f is not used, export will assume the names are variables. |
-n | Named variables (or functions, with -f) will no longer be exported. Equivalent to using the command declare +x name. |
-p | Display a list of all variables and functions marked for export. |
Examples
The following series of commands will illustrate how variables are passed to a child process in bash, with and without export. First, set the value of a new variable named myvar:
myvar="This variable is defined."
Verify that it is set, and that it has a value in the current shell context:
echo $myvar
This variable is defined.
Create a new shell context by running bash at the bash command prompt:
bash
Now you have a new bash shell, which is running inside your original bash session. The variable myvar has no value in this context, because it was not passed from the original shell:
echo $myvar
(Only a newline is printed, because myvar has no value.)
Now, exit this shell, which returns you the original bash session:
exit
Here, myvar still has a value:
echo $myvar
This variable is defined.
Now, start a new bash session again — but this time, export the variable first:
export myvar
bash
This time, the value of myvar is passed to the new shell:
echo $myvar
This variable is defined.
Great. Exit the subshell:
exit
Now let's verify that it works with functions, too.
Create a new shell function, myfunc. You can define a bash shell function by placing parentheses after the function name, and then a compound command. A compound command can take different forms; here, we will enclose it in curly braces, with a semicolon after each command, including the last one:
myfunc () { echo "This function is defined."; }
Now myfunc is a command name you can run in the current shell:
myfunc
This function is defined.
To pass this function to any child processes, use export -f:
export -f myfunc
You can verify that it is passed by starting bash in a child process, and running myfunc:
bash
myfunc
This function is defined.
Hopefully these examples help you better understand how export is useful, and give you a better understanding of how bash creates new processes.
Don't forget you can always check what variables and functions will be exported to child processes by running export -p:
export -p
(a really long list of what gets exported from the current shell)
Related commands
declare — Set or view the values and attributes of bash variables and functions.
exec — Destroy the current shell and replace it with a new process.