Boolean
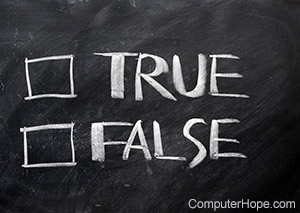
In computer science, a boolean or bool is a data type with two possible values: true or false. It is named after the English mathematician and logician George Boole, whose algebraic and logical systems are used in all modern digital computers.
Boolean is pronounced BOOL-ee-an. The word "Boolean" should only be capitalized in reference to Boolean logic or Boolean algebra. With the data type in computer programming, the word "boolean" is properly spelled with a lowercase b.
Boolean operator examples
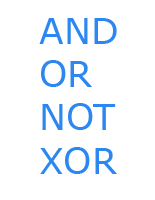
A boolean operator, or logical operator, consists of operators such as AND, OR, NOT, NOR, NAND, and XOR. These operators are used with conditional statements in programming, search engines, algorithms, and formulas.
Below is an example chart that helps explain the Boolean operations even more by detailing each of the different Boolean situations.
Example data = "Computer Hope is a location to find free computer help and information."
Boolean | Value_1 | Value_2 | Explanation | Results |
---|---|---|---|---|
AND | Free | Help | Because the above example data contains both "free" and "help" the results would be TRUE. | TRUE |
AND | Expensive | Help | Although the above example does have "help," it does not contain "expensive," which makes the result FALSE. | FALSE |
OR | Free | Help | The above example data has both "free" and "help," but the OR Boolean only requires one or the other, which makes this TRUE. | TRUE |
OR | Expensive | Help | Although "expensive" is not in the example data, it still does contain "help," which makes the TRUE. | TRUE |
NOT | Free | The above example does contain "free," which makes the result FALSE. | FALSE | |
NOT | Expensive | The above example does not contain "expensive," which makes the result TRUE. | TRUE | |
XOR | Free | Help | The above example contains both "free" and "help," the XOR Boolean only requires one or the other, but not both, making this FALSE. | FALSE |
XOR | Expensive | Help | The above example does not contain "expensive" but does contain "help," which makes this TRUE. | TRUE |
For a detailed explanation of how each of these operations works in hardware and software, see our logic operations overview.
What is a boolean data type?
In computer science, a boolean data type is any data type of true or false value, yes or no value, or on or off (1 or 0) value. By default, the boolean data type is set to false. In some programming languages, such as Perl, there is no special boolean data type. However, when using if it returns as either true or false.
Booleans used in programming
In programming, a boolean can be used with conditional statements (e.g., if statement), as shown in the following example using Perl.
use strict; my ($name, $password); print "\nName: "; chomp($name = <STDIN>); print "\nPassword: "; chomp($password = <STDIN>); if (($name eq "bob") && ($password eq "example")) { print "Success\n"; } else { print "Fail\n"; die; }
The above if statement looks for a username equal to "bob" and password equal to "example". If either the name or password is not correct, the program prints "Fail" and terminates.
Ampersand, Bitwise operators, Computer acronyms, Conditional statement, Data structure, Data type, False, Operator, Programming terms, True, XOR